최적화 테스트(Job,Burst)
2024. 8. 13. 23:19ㆍPublic/Unity
기본 가상클래스
using System.Collections;
using System.Collections.Generic;
using UnityEditor.Rendering;
using UnityEngine;
public abstract class CreateTest : MonoBehaviour
{
//테스트할 객체
public class Entity
{
public Entity(GameObject obj=null, Transform transform=null, float speed=0f)
{
_obj = obj;
_transform = transform;
_moveSpeed = speed;
}
public GameObject _obj;
public Transform _transform;
public float _moveSpeed;
}
[SerializeField] protected int DENSITY = 50;
[SerializeField] protected float _sineValue = 2f;
[SerializeField] protected Transform _prefab;
[SerializeField] protected float _sineSpeed = 2f;
protected List<Entity> _prefabList = new List<Entity>();
[SerializeField] protected Vector3 _fieldSize;
protected Vector3 _cellSize;
protected Vector2[,] _objectEntities;
protected Vector3 _startPosition;
private void Start()
{
Initialized();
CreatePrefabs();
}
void Update()
{
UpdatePrefabs();
}
protected abstract void Initialized();
protected abstract void CreatePrefabs();
protected abstract void UpdatePrefabs();
}
가장 기본(최적화X)
using System.Collections;
using System.Collections.Generic;
using Unity.Mathematics;
using UnityEngine;
public class NormalTest : CreateTest
{
protected override void CreatePrefabs()
{
for (int i = 0; i < _objectEntities.GetLength(0); i++)
for (int j = 0; j < _objectEntities.GetLength(1); j++)
{
Transform obj = Instantiate(_prefab, this.transform);
obj.localPosition = new Vector3
(
i * _cellSize.x + _startPosition.x,
j * _cellSize.y + _startPosition.y,
0f
) + new Vector3(UnityEngine.Random.Range(-0.5f, 0.5f), UnityEngine.Random.Range(-0.5f, 0.5f), 0f);
_objectEntities[i, j] = obj.transform.position;
Entity entity = new Entity(obj.gameObject, obj, UnityEngine.Random.Range(-2,3f));
_prefabList.Add(entity);
}
}
protected override void Initialized()
{
_startPosition = -_fieldSize * 0.5f;
_cellSize = new Vector3(_fieldSize.x / DENSITY, _fieldSize.y / DENSITY, _fieldSize.z / DENSITY);
_objectEntities = new Vector2[DENSITY, DENSITY];
}
protected override void UpdatePrefabs()
{
foreach (var entity in _prefabList)
{
Vector3 pos = entity._transform.position;
float speed = Time.deltaTime * entity._moveSpeed;
pos.y += Mathf.Sin(Time.time) * _sineValue * speed;
entity._transform.localPosition = pos;
float value = 0f;
for (int i = 0; i < 1000; i++)
{
value = math.exp10(math.sqrt(value));
}
}
}
}
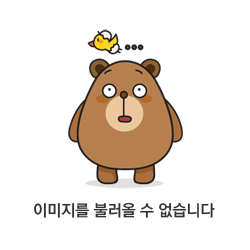
Job System 활용
using JetBrains.Annotations;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using Unity.Collections;
using Unity.Mathematics;
using Unity.Jobs;
using Random = UnityEngine.Random;
using Unity.Burst;
public class JobTest : CreateTest
{
public struct CreateJob : IJobParallelFor
{
public NativeArray<float3> _localPositions;
public NativeArray<float> _moveSpeed;
public float _time;
public float _deltaTime;
public float _sineValue;
public void Execute(int index)
{
float3 pos = _localPositions[index];
float speed = _deltaTime * _moveSpeed[index];
pos.y += math.sin(_time) * _sineValue * speed;
_localPositions[index] = pos;
float value = 0f;
for(int i = 0;i < 1000; i++)
{
value = math.exp10(math.sqrt(value));
}
}
}
protected override void CreatePrefabs()
{
for (int i = 0; i < _objectEntities.GetLength(0); i++)
for (int j = 0; j < _objectEntities.GetLength(1); j++)
{
Transform obj = Instantiate(_prefab, this.transform);
obj.localPosition = new Vector3
(
i * _cellSize.x + _startPosition.x,
j * _cellSize.y + _startPosition.y,
0f
) + new Vector3(Random.Range(-0.5f, 0.5f), Random.Range(-0.5f, 0.5f), 0f);
_objectEntities[i, j] = obj.transform.position;
Entity entity = new Entity(obj.gameObject, obj, Random.Range(-2, 3f));
_prefabList.Add(entity);
}
}
protected override void Initialized()
{
_startPosition = -_fieldSize * 0.5f;
_cellSize = new Vector3(_fieldSize.x / DENSITY, _fieldSize.y / DENSITY, _fieldSize.z / DENSITY);
_objectEntities = new Vector2[DENSITY, DENSITY];
}
protected override void UpdatePrefabs()
{
NativeArray<float3> positions = new NativeArray<float3>(_prefabList.Count, Allocator.TempJob);
NativeArray<float> speed = new NativeArray<float>(_prefabList.Count, Allocator.TempJob);
for (int i = 0; i < _prefabList.Count; i++)
{
positions[i] = _prefabList[i]._transform.position;
speed[i] = _prefabList[i]._moveSpeed;
}
CreateJob job = new CreateJob
{
_localPositions = positions,
_moveSpeed = speed,
_time = Time.time,
_deltaTime = Time.deltaTime,
_sineValue = _sineValue,
};
JobHandle handle = job.Schedule(_prefabList.Count,100);
handle.Complete();
for(int i =0; i < _prefabList.Count; i++)
{
Vector3 pos = positions[i];
_prefabList[i]._transform.localPosition = pos;
}
positions.Dispose();
speed.Dispose();
}
}
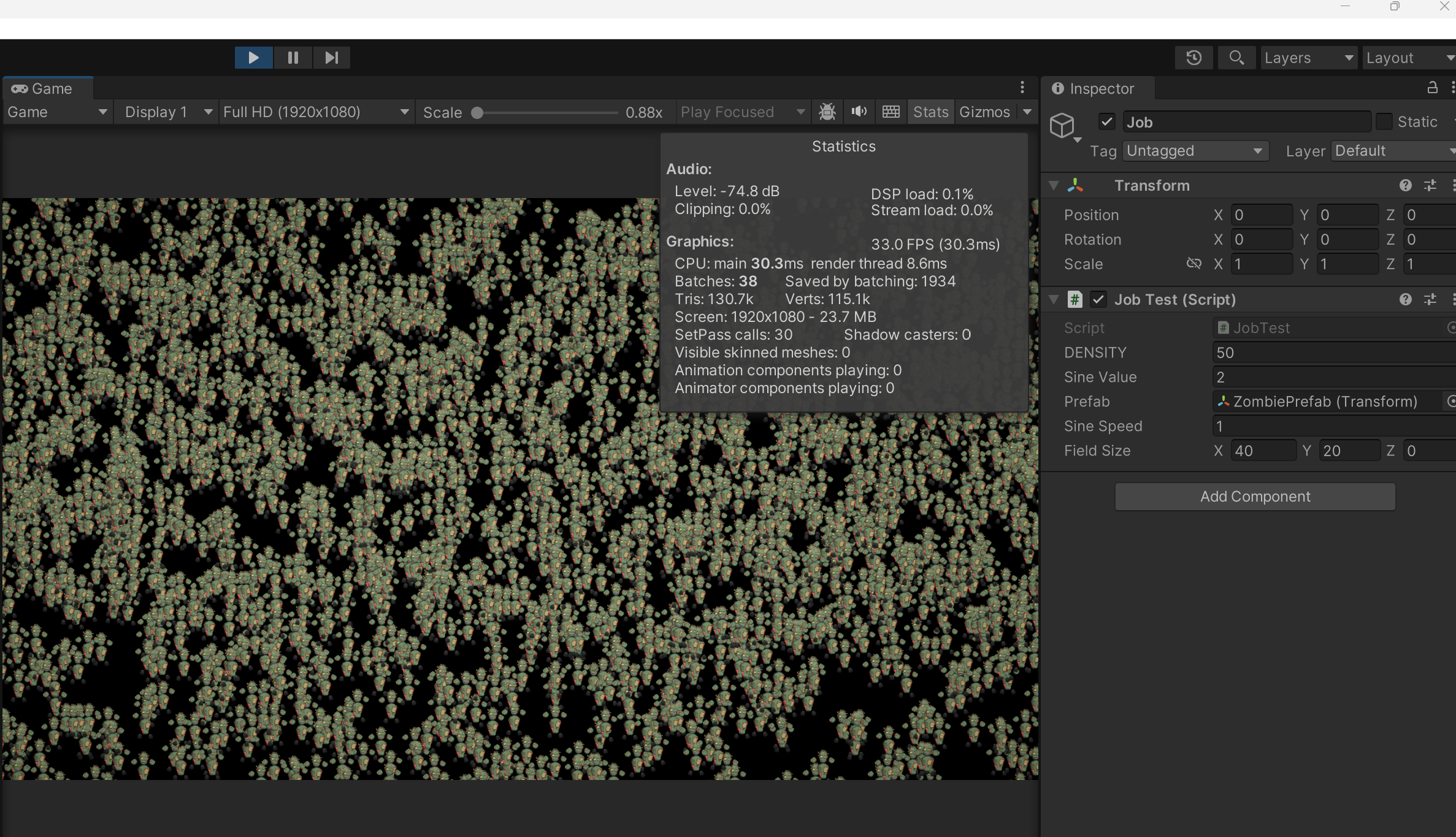
버스트 컴파일 사용
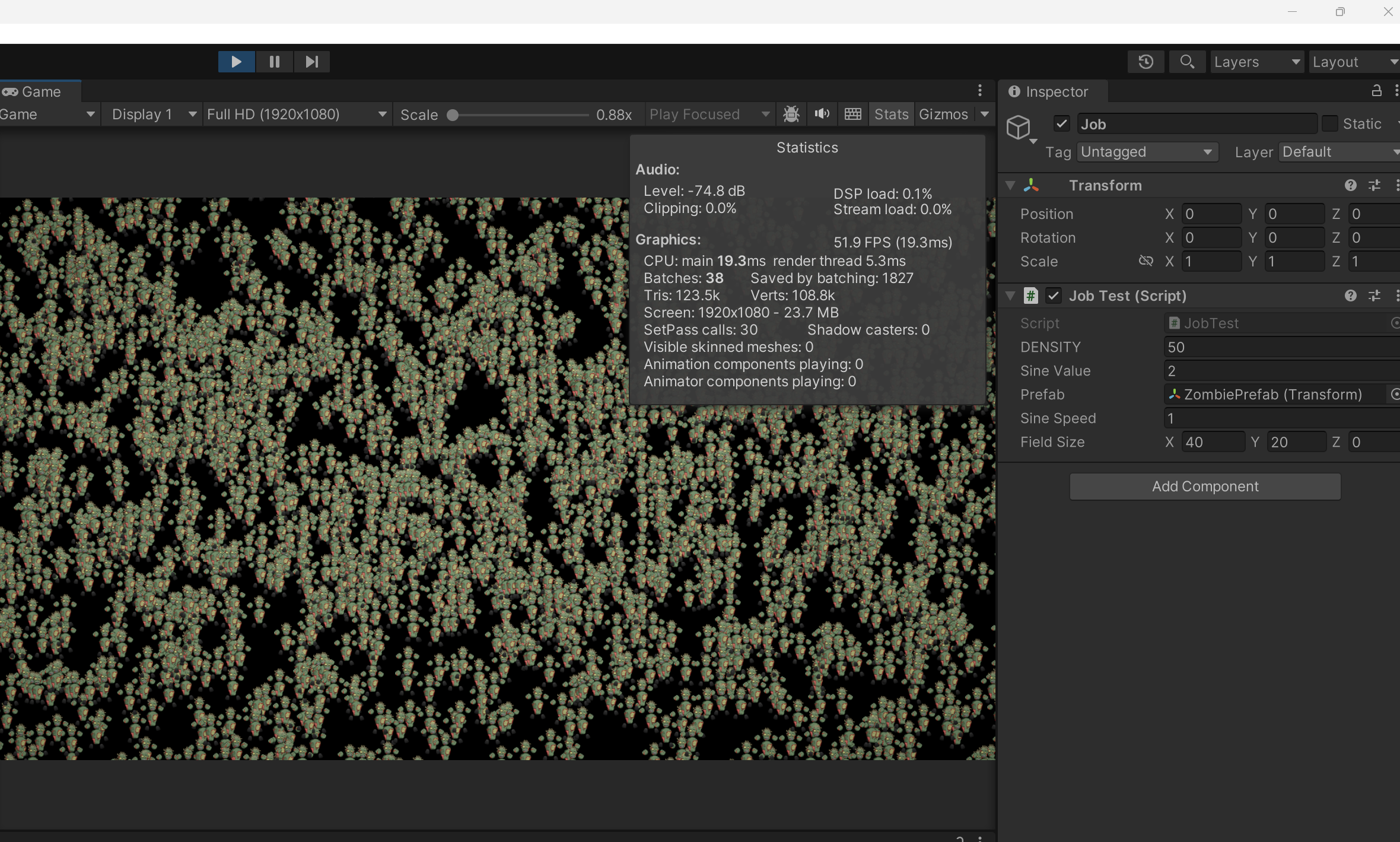
'Public > Unity' 카테고리의 다른 글
CustomTMP_Flip (0) | 2023.10.09 |
---|---|
SRP 정리 (0) | 2023.09.12 |
JungUtil(MyCustomUtil) (0) | 2023.09.11 |
Custom SRP (진행중) (0) | 2023.09.10 |
Unity Tip (0) | 2023.09.04 |